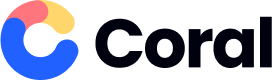
Coral is a thoroughly developed design system widely adopted by developers and designers for creating beautiful and user-friendly Sea internal products.
Copyright © 2018-2025 Sea Labs
Prop name | Type | Default | Description |
---|---|---|---|
accept | string | string[] | ||
allowClear | boolean | ||
as | any | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. | |
containerSize | number | ||
disabled | boolean | ||
files | BaseFile[] | ||
getItemProps | (file: BaseFile) => Partial<UploadItemProps> | ||
initialFiles | BaseFile[] | [] | |
inputProps | UploadInputProps | ||
maxSize | number | 2 * MB | By default is 2 * 1024 * 1024. |
minSize | number | ||
onChange | (files: BaseFile[]) => void | ||
onDrop | <T extends File>(acceptedFiles: T[], rejectedFiles: T[], event: DropEvent) => void | When the files selected are rejected (due to maxSize, minSize, accepts, etc), you can get the rejectedFiles from the second parameter. | |
placeholder | ReactNode | ||
type | UploadItemTypes | gallery | |
variant | UploadVariants | basic |
Prop name | Type | Default | Description |
---|---|---|---|
as | any | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. | |
disabled | boolean | Equivalent to button's disabled property. | |
keyboardClickKeys | string[] | ||
onBlur | FocusEventHandler<HTMLDivElement> | ||
onClick | MouseEventHandler<HTMLDivElement> | ||
onKeyDown | KeyboardEventHandler<HTMLDivElement> | ||
onKeyUp | KeyboardEventHandler<HTMLDivElement> | ||
onMouseDown | MouseEventHandler<HTMLDivElement> | ||
onMouseLeave | MouseEventHandler<HTMLDivElement> | ||
onMouseUp | MouseEventHandler<HTMLDivElement> | ||
onRelease | EventHandler<SyntheticEvent<HTMLDivElement, Event>> | Callback that's triggered after click i.e. mouseup and keyup of enter or space. | |
onTouchEnd | TouchEventHandler<HTMLDivElement> | ||
onTouchStart | TouchEventHandler<HTMLDivElement> |
Prop name | Type | Default | Description |
---|---|---|---|
as | any | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. | |
columns | number | 3 | |
containerWidth | number | 512 |
Prop name | Type | Default | Description |
---|---|---|---|
as | any | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. | |
containerSize | number | ||
inputProps | HTMLAttributes<HTMLInputElement> | ||
invalid | boolean | ||
onDrop | <T extends File>(acceptedFiles: T[], rejectedFiles: T[], event: DropEvent) => void | When the files selected are rejected (due to maxSize, minSize, accepts, etc), you can get the rejectedFiles from the second parameter. | |
placeholder | ReactNode | <UploadPlaceholder /> |
Prop name | Type | Default | Description |
---|---|---|---|
as | any | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. | |
containerHeight | number | ||
containerProps | DivProps | ||
containerWidth | number | ||
deleteButtonProps | IconButtonProps | ||
detailProps | DivProps | ||
extensionProps | DivProps | ||
file | BaseFile | ||
icon | ReactNode | ||
iconProps | DivProps | ||
invalid | boolean | ||
message | ReactNode | ||
messageProps | DivProps | ||
nameProps | DivProps | ||
nameWrapperProps | DivProps | ||
onDelete | (e: SyntheticEvent<HTMLDivElement, Event>) => void | ||
onPreview | (e: SyntheticEvent<HTMLDivElement, Event>) => void | ||
previewButtonProps | IconButtonProps | ||
progress | number | ||
sizeProps | DivProps |
Prop name | Type | Default | Description |
---|---|---|---|
as | any | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. | |
file | File | ||
invalid | boolean | ||
progress | number |
Prop name | Type | Default | Description |
---|---|---|---|
as | any | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. | |
containerHeight | number | ||
containerProps | DivProps | ||
containerWidth | number | ||
deleteButtonProps | IconButtonProps | ||
detailProps | DivProps | ||
extensionProps | DivProps | ||
file | File | ||
icon | ReactNode | ||
iconProps | DivProps | ||
invalid | boolean | ||
message | ReactNode | ||
messageProps | DivProps | ||
nameProps | DivProps | ||
nameWrapperProps | DivProps | ||
onDelete | (e: SyntheticEvent<HTMLDivElement, Event>) => void | ||
onPreview | (e: SyntheticEvent<HTMLDivElement, Event>) => void | ||
previewButtonProps | IconButtonProps | ||
progress | number | ||
sizeProps | DivProps |
Prop name | Type | Default | Description |
---|---|---|---|
as | any | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. | |
containerHeight | number | ||
containerProps | DivProps | ||
containerWidth | number | ||
deleteButtonProps | IconButtonProps | ||
detailProps | DivProps | ||
extensionProps | DivProps | ||
file | BaseFile | ||
icon | ReactNode | ||
iconProps | DivProps | ||
invalid | boolean | ||
message | ReactNode | ||
messageProps | DivProps | ||
nameProps | DivProps | ||
nameWrapperProps | DivProps | ||
onDelete | (e: SyntheticEvent<HTMLDivElement, Event>) => void | ||
onPreview | (e: SyntheticEvent<HTMLDivElement, Event>) => void | ||
previewButtonProps | IconButtonProps | ||
progress | number | ||
sizeProps | DivProps |
Prop name | Type | Default | Description |
---|---|---|---|
as | any | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. | |
description | ReactNode | ||
descriptionProps | DivProps | ||
image | string | ||
text | ReactNode | ||
textProps | DivProps |
Prop name | Type | Default | Description |
---|---|---|---|
as | any | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. | |
image | string | ||
text | ReactNode | ||
textProps | DivProps |
Copyright © 2018-2025 Sea Labs