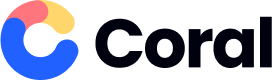
Coral is a thoroughly developed design system widely adopted by developers and designers for creating beautiful and user-friendly Sea internal products.
Copyright © 2018-2025 Sea Labs
Prop name | Type | Default | Description |
---|---|---|---|
align | TextAlign | ||
as | any | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. | |
cellProps | (record: any, index: number) => TableCellProps | ||
depth | number | 0 | |
ellipsis | boolean | ||
hidden | boolean | ||
index | number | ||
loading | boolean | Determines whether the Column is in a loading state, showing skeleton placeholders. | |
record | any | ||
render | (record: any, index: number) => ReactNode | ||
renderLoading | (record: any, index: number) => ReactNode | () => <Skeleton variant="rect" width="70%" height="100%" /> | Customize the component to render on loading state |
sticky | ColumnStickyPositions | ColumnStickyPositions[] | { left?: number; right?: number; } | ||
title | ReactNode | ||
titleProps | TableCellProps |
Prop name | Type | Default | Description |
---|---|---|---|
align | TextAlign | ||
as | any | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. | |
cellProps | (record: any, index: number) => TableCellProps | ||
depth | number | ||
ellipsis | boolean | ||
hidden | boolean | ||
index | number | ||
isDisabled | (row: any, index: number) => boolean | ||
loading | boolean | Determines whether the Column is in a loading state, showing skeleton placeholders. | |
onChange | (value: RowKey[], valueObjects: any[]) => void | valueObjects will be returned based on the object data ever received by the TableData component.undefined will be returned in place of the object if TableData component never received object with the particular key. | |
onDeselect | SelectionCallback<RowKey> | ||
onDeselectAll | AllSelectionCallback<RowKey> | ||
onSelect | SelectionCallback<RowKey> | ||
onSelectAll | AllSelectionCallback<RowKey> | ||
record | any | ||
render | (props: RenderParams<any>) => ReactNode | <T extends unknown>({ children }: RenderParams<T>) => children | |
renderLoading | (record: any, index: number) => ReactNode | Customize the component to render on loading state | |
renderTitle | (props: { children: ReactElement<any, string | JSXElementConstructor<any>>; }) => ReactNode | ({ children }: { children: ReactElement }) => children | |
sticky | ColumnStickyPositions | ColumnStickyPositions[] | { left?: number; right?: number; } | ||
titleProps | TableCellProps | ||
value | RowKey[] |
Prop name | Type | Default | Description |
---|---|---|---|
as | any | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. | |
bodyProps | TableBodyProps | ||
data | any[] | ||
emptyPlaceholder | ReactNode | <Result image={<EmptyBox size={64} />} description="No data" /> | |
expandedRows | RowKey[] | ||
footer | ReactNode | ||
headProps | TableHeadProps | ||
headRowProps | TableRowProps | ||
isExpandable | (record: any, index: number) => boolean | ||
loading | boolean | Determines whether the table is in a loading state, showing skeleton placeholders. | |
loadingRowsCount | number | 5 | Specifies the number of rows to display in the loading state. |
onChangeExpandedRows | (expandedRows: RowKey[], valueObjects: any[]) => void | ||
renderExpandableRow | RenderExpandableRow<any> | ||
renderRow | RenderRow<any> | ||
rowKey | (record: any, index: number) => RowKey | ||
tableProps | TableProps |
Prop name | Type | Default | Description |
---|---|---|---|
as | any | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. | |
colorType | ColorTypes | Set color based on theme. If color is specified, that prop is used instead of this. | |
disabled | boolean | Equivalent to button's disabled property. | |
isExpandable | boolean | ||
keyboardClickKeys | string[] | ||
loading | boolean | ||
onBlur | FocusEventHandler<HTMLDivElement> | ||
onClick | MouseEventHandler<HTMLDivElement> | ||
onKeyDown | KeyboardEventHandler<HTMLDivElement> | ||
onKeyUp | KeyboardEventHandler<HTMLDivElement> | ||
onMouseDown | MouseEventHandler<HTMLDivElement> | ||
onMouseLeave | MouseEventHandler<HTMLDivElement> | ||
onMouseUp | MouseEventHandler<HTMLDivElement> | ||
onRelease | EventHandler<SyntheticEvent<HTMLDivElement, Event>> | Callback that's triggered after click i.e. mouseup and keyup of enter or space. | |
onTouchEnd | TouchEventHandler<HTMLDivElement> | ||
onTouchStart | TouchEventHandler<HTMLDivElement> | ||
radius | string | ||
renderIcon | (props: { depth: number; isExpanded: boolean; }) => ReactNode | ||
size | IconButtonSizes | small | |
tooltip | ReactNode | ||
tooltipProps | Omit<TooltipProps, "title" | "children"> | ||
variant | IconButtonVariants |
Prop name | Type | Default | Description |
---|---|---|---|
alignment | PaginationAlignments | Controls how the pagination items (like pages or page text) are aligned in the main container.
| |
as | any | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. | |
children | ReactNode | React children that can be used to override or extend the default pagination items. For example, you can pass <PaginationList /> or <PaginationPageText /> here. | |
current | number | The controlled current page number (1-based). If used, initialCurrent is ignored and the component becomes controlled. | |
initialCurrent | number | The initial (uncontrolled) current page number. Defaults to 1. | |
initialNavSpan | number | ||
initialPageSize | number | 20 | |
leftContainerProps | DivProps | ||
leftElement | ReactNode | Defaults to showing total pages and selected items for non-minimal variants. | |
navSpan | number | The controlled number of pagination elements to show (e.g., 7 shows a maximum of 7 page buttons). Must be an odd number greater than 3 for best display. | |
numSelected | number | (Optional) Number of selected items (out of totalRow). Used by default to display information like "x selected". | |
onChange | (page: number) => void | A callback that fires when the current page changes (e.g., user navigates to a new page). Receives the new page number as its argument. | |
onNavSpanChange | (navSpan: number) => void | ||
onPageSizeChange | (pageSize: number) => void | ||
pageSize | number | The controlled page size (number of items per page). If used, initialPageSize is ignored and the component becomes controlled. | |
rightContainerProps | DivProps | ||
rightElement | ReactNode | Defaults to showing page navigation for non-minimal variants. | |
sizes | number[] | Selectable page sizes. | |
totalRow | number | The total number of rows (items) across all pages. This is used to calculate the number of pages. | |
variant | PaginationVariants | outlined | The variant of pagination style to use.
|
Prop name | Type | Default | Description |
---|---|---|---|
as | any | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. | |
index | number | ||
isSelected | boolean |
Copyright © 2018-2025 Sea Labs