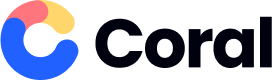
Coral is a thoroughly developed design system widely adopted by developers and designers for creating beautiful and user-friendly Sea internal products.
Copyright © 2018-2025 Sea Labs
Prop name | Type | Default | Description |
---|---|---|---|
as | any | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. | |
date | Dayjs | ||
dateFooter | ReactNode | ||
dateHeader | ReactNode | ||
dateViewProps | ViewProps | ||
immediate | boolean | ||
initialDate | Dayjs | dayjs() | |
initialMode | CalendarModes | date | |
mode | CalendarModes | ||
monthFooter | ReactNode | ||
monthHeader | ReactNode | ||
monthViewProps | ViewProps | ||
onDateChange | (date: Dayjs) => void | ||
onModeChange | (mode: CalendarModes) => void | ||
onPreviousModeChange | (mode: CalendarModes) => void | ||
previousMode | CalendarModes | ||
renderDate | (props: RenderCalendarDateOptions, defaultCell: ReactElement<CalendarDateCellProps, any>) => ReactNode | ||
renderDay | (props: RenderDayOptions, defaultCell: ReactElement<RenderDayOptions, any>) => ReactNode | ||
renderMonth | (props: RenderCalendarMonthOptions, defaultCell: ReactElement<CalendarMonthCellProps, any>) => ReactNode | ||
renderYear | (props: RenderCalendarYearOptions, defaultCell: ReactElement<CalendarYearCellProps, any>) => ReactNode | ||
startOfWeek | Day | 0 | Accepts numbers from 0 (Sunday) to 6 (Saturday). |
today | Dayjs | dayjs() | |
yearFooter | ReactNode | ||
yearHeader | ReactNode | ||
yearViewProps | ViewProps |
Prop name | Type | Default | Description |
---|---|---|---|
as | any | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. | |
date | Dayjs | ||
disableGrid | boolean | ||
disabled | boolean | Equivalent to button's disabled property. | |
keyboardClickKeys | string[] | ||
onBlur | FocusEventHandler<HTMLDivElement> | ||
onClick | MouseEventHandler<HTMLDivElement> | ||
onGridFocus | () => void | ||
onKeyDown | KeyboardEventHandler<HTMLDivElement> | ||
onKeyUp | KeyboardEventHandler<HTMLDivElement> | ||
onMouseDown | MouseEventHandler<HTMLDivElement> | ||
onMouseLeave | MouseEventHandler<HTMLDivElement> | ||
onMouseUp | MouseEventHandler<HTMLDivElement> | ||
onRelease | EventHandler<SyntheticEvent<HTMLDivElement, Event>> | Callback that's triggered after click i.e. mouseup and keyup of enter or space. | |
onTouchEnd | TouchEventHandler<HTMLDivElement> | ||
onTouchStart | TouchEventHandler<HTMLDivElement> | ||
preventScroll | boolean | ||
range | Range | ||
startOfWeek | Day | 0 | |
today | Dayjs | dayjs() | |
variant | CellVariants |
Prop name | Type | Default | Description |
---|---|---|---|
as | any | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. | |
month | number | ||
renderDate | (props: RenderDateOptions, defaultCell: ReactElement<RenderDateOptions, any>) => ReactNode | (props: RenderDateOptions) => <DateCell {...props} /> | |
renderDay | (props: RenderDayOptions, defaultCell: ReactElement<RenderDayOptions, any>) => ReactNode | ({ day }: RenderDayOptions) => <DayCell day={day} /> | |
startOfWeek | Day | ||
year | number |
Prop name | Type | Default | Description |
---|---|---|---|
as | void | WebTarget | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. | |
forwardedAs | void | WebTarget | ||
theme | DefaultTheme |
Prop name | Type | Default | Description |
---|---|---|---|
as | any | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. | |
disableGrid | boolean | ||
disabled | boolean | Equivalent to button's disabled property. | |
keyboardClickKeys | string[] | ||
month | Dayjs | ||
onBlur | FocusEventHandler<HTMLDivElement> | ||
onClick | MouseEventHandler<HTMLDivElement> | ||
onGridFocus | () => void | ||
onKeyDown | KeyboardEventHandler<HTMLDivElement> | ||
onKeyUp | KeyboardEventHandler<HTMLDivElement> | ||
onMouseDown | MouseEventHandler<HTMLDivElement> | ||
onMouseLeave | MouseEventHandler<HTMLDivElement> | ||
onMouseUp | MouseEventHandler<HTMLDivElement> | ||
onRelease | EventHandler<SyntheticEvent<HTMLDivElement, Event>> | Callback that's triggered after click i.e. mouseup and keyup of enter or space. | |
onTouchEnd | TouchEventHandler<HTMLDivElement> | ||
onTouchStart | TouchEventHandler<HTMLDivElement> | ||
preventScroll | boolean | ||
range | Range | ||
today | Dayjs | dayjs() | |
variant | CellVariants |
Prop name | Type | Default | Description |
---|---|---|---|
as | any | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. | |
renderMonth | (props: RenderMonthOptions, defaultCell: ReactElement<CalendarMonthCellProps, any>) => ReactNode | (props: RenderMonthOptions) => <MonthCell {...props} /> | |
year | number |
Prop name | Type | Default | Description |
---|---|---|---|
as | any | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. | |
disableGrid | boolean | ||
disabled | boolean | Equivalent to button's disabled property. | |
keyboardClickKeys | string[] | ||
onBlur | FocusEventHandler<HTMLDivElement> | ||
onClick | MouseEventHandler<HTMLDivElement> | ||
onGridFocus | () => void | ||
onKeyDown | KeyboardEventHandler<HTMLDivElement> | ||
onKeyUp | KeyboardEventHandler<HTMLDivElement> | ||
onMouseDown | MouseEventHandler<HTMLDivElement> | ||
onMouseLeave | MouseEventHandler<HTMLDivElement> | ||
onMouseUp | MouseEventHandler<HTMLDivElement> | ||
onRelease | EventHandler<SyntheticEvent<HTMLDivElement, Event>> | Callback that's triggered after click i.e. mouseup and keyup of enter or space. | |
onTouchEnd | TouchEventHandler<HTMLDivElement> | ||
onTouchStart | TouchEventHandler<HTMLDivElement> | ||
preventScroll | boolean | ||
range | Range | ||
today | Dayjs | dayjs() | |
type | YearTypes | ||
variant | CellVariants | ||
year | Dayjs |
Prop name | Type | Default | Description |
---|---|---|---|
as | any | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. | |
decade | number | ||
renderYear | (props: RenderYearOptions, defaultCell: ReactElement<CalendarYearCellProps, any>) => ReactNode | (props: RenderYearOptions) => <YearCell {...props} /> |
Copyright © 2018-2025 Sea Labs