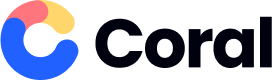
Coral is a thoroughly developed design system widely adopted by developers and designers for creating beautiful and user-friendly Sea internal products.
Copyright © 2018-2025 Sea Labs
Prop name | Type | Default | Description |
---|---|---|---|
id | string | ||
initialIsOpen | boolean | ||
initialKeyboardHighlightedIndex | number | ||
initialMouseHighlightedIndex | number | ||
initialSearchValue | string | ||
initialSelectedIndex | number | ||
initialValue | MultiSelectValue | [] | |
isOpen | boolean | ||
keyboardHighlightedIndex | number | ||
mouseHighlightedIndex | number | ||
onChange | ChangeCallback | ||
onIsOpenChange | (isOpen: boolean) => void | ||
onKeyboardHighlightedIndexChange | (index: number) => void | ||
onMouseHighlightedIndexChange | (index: number) => void | ||
onSearch | SearchCallback | ||
onSelect | ChangeCallback | Different from onChange: onSelect is triggered even if the option's value is the selected one. | |
onSelectedIndexChange | (index: number) => void | ||
searchValue | string | This is not the selected value. Rather, it's a search value on input if you use SelectSearch. | |
selectedIndex | number | ||
stateReducer | AcdcStateReducer | (state, { nextState }) => nextState | |
value | MultiSelectValue |
Prop name | Type | Default | Description |
---|---|---|---|
as | any | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. | |
clickable | boolean | ||
description | ReactNode | Description to show for capsule-large choice and filter chips. | |
disableNavigation | boolean | ||
disabled | boolean | Equivalent to button's disabled property. | |
ellipsisTooltipProps | Omit<EllipsisTooltipProps, "children"> | ||
index | number | Order index for keyboard navigation using arrow keys. By default, this prop is automatically added by the parent. | |
invalid | boolean | ||
keyboardClickKeys | string[] | ||
leftElement | ReactNode | Element to be added on the left side of the label. Equivalent to adding the element manually on the label with a certain margin. | |
onBlur | FocusEventHandler<HTMLDivElement> | ||
onClick | MouseEventHandler<HTMLDivElement> | ||
onDelete | (e: SyntheticEvent<HTMLDivElement, Event>) => void | Callback on Backspace key or delete icon is clicked. If this callback is not provided, icon is not rendered - meaning it's not deletable. | |
onKeyDown | KeyboardEventHandler<HTMLDivElement> | ||
onKeyUp | KeyboardEventHandler<HTMLDivElement> | ||
onMouseDown | MouseEventHandler<HTMLDivElement> | ||
onMouseLeave | MouseEventHandler<HTMLDivElement> | ||
onMouseUp | MouseEventHandler<HTMLDivElement> | ||
onRelease | EventHandler<SyntheticEvent<HTMLDivElement, Event>> | Callback that's triggered after click i.e. mouseup and keyup of enter or space. | |
onTouchEnd | TouchEventHandler<HTMLDivElement> | ||
onTouchStart | TouchEventHandler<HTMLDivElement> | ||
rightElement | ReactNode | Element to be added on the right side of the label. Equivalent to adding the element manually on the label with a certain margin. | |
value | SelectValue | ||
variant | ChipVariants |
Prop name | Type | Default | Description |
---|---|---|---|
active | boolean | ||
arrow | ArrowProps | ||
as | any | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. | |
closeDelay | number | ||
container | HTMLElement | ||
containerProps | InnerProps | ||
contentProps | InnerProps | ||
immediate | boolean | ||
initialActive | boolean | ||
initialImmediate | boolean | ||
maxRows | number | ||
offset | [number, number] | ||
onActiveChange | (active: boolean) => void | ||
onImmediateChange | (immediate: boolean) => void | ||
onOverflowChange | (overflow: boolean) => void | ||
openDelay | number | ||
overflow | boolean | ||
placement | "left" | "right" | "bottom" | "top" | "top-start" | "top-end" | "left-start" | "left-end" | "right-start" | "right-end" | "bottom-start" | "bottom-end" | ||
popperOptions | PopperPopperOptions | Options for floating.ui instance | |
popperUpdateKey | string | ||
renderChild | (child: any, handlers: any, ariaAttrs: any) => ReactNode | ||
springConfig | Partial<AnimationConfig> | ||
title | ReactNode | ||
titleContainerProps | HTMLAttributes<HTMLDivElement> | ||
variant | TooltipVariants |
Prop name | Type | Default | Description |
---|---|---|---|
text | string |
Prop name | Type | Default | Description |
---|---|---|---|
as | any | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. | |
badgeCount | number | ||
badgeProps | Omit<BadgeProps, "children"> | ||
colorType | ColorTypes | Set color based on theme. If color is specified, that prop is used instead of this. | |
disabled | boolean | Equivalent to button's disabled property. | |
keyboardClickKeys | string[] | [] | |
loading | boolean | ||
onBlur | FocusEventHandler<HTMLDivElement> | ||
onClick | MouseEventHandler<HTMLDivElement> | ||
onKeyDown | KeyboardEventHandler<HTMLDivElement> | ||
onKeyUp | KeyboardEventHandler<HTMLDivElement> | ||
onMouseDown | MouseEventHandler<HTMLDivElement> | ||
onMouseLeave | MouseEventHandler<HTMLDivElement> | ||
onMouseUp | MouseEventHandler<HTMLDivElement> | ||
onRelease | EventHandler<SyntheticEvent<HTMLDivElement, Event>> | Callback that's triggered after click i.e. mouseup and keyup of enter or space. | |
onTouchEnd | TouchEventHandler<HTMLDivElement> | ||
onTouchStart | TouchEventHandler<HTMLDivElement> | ||
radius | string | ||
size | IconButtonSizes | ||
tooltip | ReactNode | ||
tooltipProps | Omit<TooltipProps, "title" | "children"> | ||
variant | IconButtonVariants |
Prop name | Type | Default | Description |
---|---|---|---|
as | any | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. | |
disableChipNavigation | boolean | ||
disabled | boolean | Equivalent to button's disabled property. | |
inputChipListProps | InputChipListProps & { ref?: Ref<HTMLDivElement>; } | ||
keyboardClickKeys | string[] | [] | |
onBlur | FocusEventHandler<HTMLDivElement> | ||
onClick | MouseEventHandler<HTMLDivElement> | ||
onKeyDown | KeyboardEventHandler<HTMLDivElement> | ||
onKeyUp | KeyboardEventHandler<HTMLDivElement> | ||
onMouseDown | MouseEventHandler<HTMLDivElement> | ||
onMouseLeave | MouseEventHandler<HTMLDivElement> | ||
onMouseUp | MouseEventHandler<HTMLDivElement> | ||
onRelease | EventHandler<SyntheticEvent<HTMLDivElement, Event>> | Callback that's triggered after click i.e. mouseup and keyup of enter or space. | |
onTouchEnd | TouchEventHandler<HTMLDivElement> | ||
onTouchStart | TouchEventHandler<HTMLDivElement> | ||
placeholder | ReactNode | ||
renderChip | (val: SelectValue, i: number) => ReactNode | (val) => <MultiSelectChip key={val} value={val} /> | Renders chips per value when MultiSelect is open |
searchElement | ReactNode | <MultiSelectSearch /> |
Prop name | Type | Default | Description |
---|---|---|---|
as | any | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. | |
disableChipNavigation | boolean | ||
disabled | boolean | Equivalent to button's disabled property. | |
inputChipListProps | InputChipListProps & { ref?: Ref<HTMLDivElement>; } | ||
keyboardClickKeys | string[] | ||
label | ReactNode | ||
limit | number | 2 | |
onBlur | FocusEventHandler<HTMLDivElement> | ||
onClick | MouseEventHandler<HTMLDivElement> | ||
onKeyDown | KeyboardEventHandler<HTMLDivElement> | ||
onKeyUp | KeyboardEventHandler<HTMLDivElement> | ||
onMouseDown | MouseEventHandler<HTMLDivElement> | ||
onMouseLeave | MouseEventHandler<HTMLDivElement> | ||
onMouseUp | MouseEventHandler<HTMLDivElement> | ||
onRelease | EventHandler<SyntheticEvent<HTMLDivElement, Event>> | Callback that's triggered after click i.e. mouseup and keyup of enter or space. | |
onTouchEnd | TouchEventHandler<HTMLDivElement> | ||
onTouchStart | TouchEventHandler<HTMLDivElement> | ||
placeholder | ReactNode | ||
renderChip | (val: SelectValue, i: number) => ReactNode | Renders chips per value when MultiSelect is open | |
searchElement | ReactNode | ||
tooltipProps | Omit<TooltipProps, "title" | "children"> & { children?: ReactNode; title?: ReactNode; } |
Prop name | Type | Default | Description |
---|---|---|---|
as | any | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. | |
contentProps | ContentProps | {} | |
disableChipNavigation | boolean | ||
disabled | boolean | Equivalent to button's disabled property. | |
inputChipListProps | InputChipListProps & { ref?: Ref<HTMLDivElement>; } | ||
keyboardClickKeys | string[] | ||
onBlur | FocusEventHandler<HTMLDivElement> | ||
onClick | MouseEventHandler<HTMLDivElement> | ||
onKeyDown | KeyboardEventHandler<HTMLDivElement> | ||
onKeyUp | KeyboardEventHandler<HTMLDivElement> | ||
onMouseDown | MouseEventHandler<HTMLDivElement> | ||
onMouseLeave | MouseEventHandler<HTMLDivElement> | ||
onMouseUp | MouseEventHandler<HTMLDivElement> | ||
onRelease | EventHandler<SyntheticEvent<HTMLDivElement, Event>> | Callback that's triggered after click i.e. mouseup and keyup of enter or space. | |
onTouchEnd | TouchEventHandler<HTMLDivElement> | ||
onTouchStart | TouchEventHandler<HTMLDivElement> | ||
placeholder | ReactNode | ||
renderChip | (val: SelectValue, i: number) => ReactNode | Renders chips per value when MultiSelect is open | |
renderText | (val: MultiSelectValue) => ReactNode | Renders displayed text when MultiSelect is closed | |
searchElement | ReactNode | ||
tooltipProps | Omit<EllipsisTooltipProps, "children"> & { children?: ReactNode; } |
Prop name | Type | Default | Description |
---|---|---|---|
acdcScrollerPropsOptions | UseAcdcScrollerPropsOptions | Options for the useAcdcScrollerProps hook | |
as | any | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. | |
container | HTMLElement | ||
containerProps | DivProps | ||
elevationLevel | number | ||
emptyPlaceholder | ReactNode | ||
footer | ReactNode | ||
header | ReactElement<any, any> | ||
immediate | boolean | ||
placement | "left" | "right" | "bottom" | "top" | "top-start" | "top-end" | "left-start" | "left-end" | "right-start" | "right-end" | "bottom-start" | "bottom-end" | ||
popperReference | HTMLElement | ||
popperUpdateKey | string | ||
width | SelectListWidth |
Prop name | Type | Default | Description |
---|---|---|---|
as | any | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. |
Prop name | Type | Default | Description |
---|---|---|---|
as | any | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. | |
autoComplete | "on" | "off" | "nope" | ||
disabled | boolean | ||
fullWidth | boolean | width: 100% is too troublesome to handle with custom css because of the nesting | |
invalid | boolean | ||
leftElement | ReactNode | ||
leftElementContainerProps | HTMLAttributes<HTMLDivElement> & { ref?: MutableRefObject<HTMLDivElement>; } | ||
onChange | (e: ChangeEvent<HTMLInputElement>) => void | ||
onTextChange | (val: string) => void | ||
placeholder | ReactNode | ||
readOnly | boolean | ||
rightElement | ReactNode | ||
rightElementContainerProps | HTMLAttributes<HTMLDivElement> & { ref?: MutableRefObject<HTMLDivElement>; } | ||
type | "number" | "text" | "password" | ||
wrapperProps | WrapperProps |
Prop name | Type | Default | Description |
---|---|---|---|
as | any | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. | |
checkboxIconProp | CheckboxIconProps | ||
disabled | boolean | ||
index | number | ||
value | SelectValue |
Prop name | Type | Default | Description |
---|---|---|---|
as | any | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. |
Prop name | Type | Default | Description |
---|---|---|---|
acdcScrollerPropsOptions | UseAcdcScrollerPropsOptions | Options for the useAcdcScrollerProps hook | |
as | any | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. | |
container | HTMLElement | ||
containerProps | DivProps | ||
elevationLevel | number | ||
emptyPlaceholder | ReactNode | ||
estimatedItemSize | number | ||
footer | ReactNode | ||
header | ReactElement<any, any> | ||
immediate | boolean | ||
innerProps | HTMLAttributes<HTMLUListElement> & { as?: any; ref?: Ref<HTMLUListElement>; } | ||
itemSize | (index: number) => number | ||
overscanCount | number | ||
placement | "left" | "right" | "bottom" | "top" | "top-start" | "top-end" | "left-start" | "left-end" | "right-start" | "right-end" | "bottom-start" | "bottom-end" | ||
popperReference | HTMLElement | ||
popperUpdateKey | string | ||
width | SelectListWidth |
Copyright © 2018-2025 Sea Labs