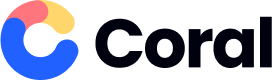
Coral is a thoroughly developed design system widely adopted by developers and designers for creating beautiful and user-friendly Sea internal products.
Copyright © 2018-2025 Sea Labs
Prop name | Type | Default | Description |
---|---|---|---|
as | any | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. | |
hourStep | number | 1 | |
initialValue | Dayjs | ||
isHourDisabled | (hour: number) => boolean | () => false | |
isMinuteDisabled | (minute: number, hour: number) => boolean | () => false | |
isSecondDisabled | (second: number, minute: number, hour: number) => boolean | () => false | |
minuteStep | number | 1 | |
now | Dayjs | dayjs() | |
onChange | (value: Dayjs) => void | ||
secondStep | number | 1 | |
value | Dayjs |
Prop name | Type | Default | Description |
---|---|---|---|
disabled | boolean | Disable input, time selection, and opening popup. | |
displayTime | (value: Dayjs) => string | (value: Dayjs | null) => (value ? value.format(timeFormat) : '') | Callback to convert value to inputValue |
hourStep | number | 1 | |
initialIsOpen | boolean | Uncontrolled of popup open state. | |
initialValue | Dayjs | ||
inputElement | ReactElement<any, any> | <TimePickerInput /> | Customizing input. Normally, how you use this prop is passing DatePickerInput with some additional props. |
inputValue | string | Controlled input value. You can think of this prop as text representation of value using dateFormat. | |
isHourDisabled | (hour: number) => boolean | () => false | Whether a certain time cannot be selected. This prop is not only used to disable times on the spinbuttons, but also for input not to trigger selection when input is a valid time but disabled. |
isMinuteDisabled | (minute: number, hour: number) => boolean | () => false | |
isOpen | boolean | Controlled of popup open state. | |
isSecondDisabled | (second: number, minute: number, hour: number) => boolean | () => false | |
minuteStep | number | 1 | |
now | Dayjs | dayjs() | Useful for consistent testing, default to dayjs() |
onChange | (value: Dayjs) => void | ||
onInputChange | (inputValue: string) => void | Callback when input value changes.Generally, it's triggered by: (1) Typing on the input. (2) Blur or clicking away from input, which will reset to selected value. (3) A time is selected. | |
onIsOpenChange | (isOpen: boolean) => void | Callback when popup open state changes.The event that triggers opening the popup is the same as Balloon component's - either click or keyboard arrow down. Closing is triggered when a date is selected. | |
parseTime | (inputValue: string) => Dayjs | (value: string) => dayjs(value, timeFormat) | Callback to convert inputValue to value |
popupElement | ReactElement<any, any> | <TimePickerPopup /> | Customizing popup. Normally, how you use this prop is passing DatePickerPopup with some additional props. |
secondStep | number | 1 | |
timeFormat | string | HH:mm | Dayjs format to display from selected time, and parse input to autoselect time when the parse is valid. |
value | Dayjs |
Prop name | Type | Default | Description |
---|---|---|---|
as | any | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. | |
header | ReactNode | Hour | |
headerProps | HeaderProps | ||
index | number | ||
scrollerProps | TimePickerScrollerProps & { ref?: Ref<HTMLDivElement>; } |
Prop name | Type | Default | Description |
---|---|---|---|
as | any | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. | |
clearable | boolean | ||
colorType | ColorTypes | Set color based on theme. If color is specified, that prop is used instead of this. | |
disabled | boolean | Equivalent to button's disabled property. | |
keyboardClickKeys | string[] | ||
loading | boolean | ||
onBlur | FocusEventHandler<HTMLDivElement> | ||
onClick | MouseEventHandler<HTMLDivElement> | ||
onKeyDown | KeyboardEventHandler<HTMLDivElement> | ||
onKeyUp | KeyboardEventHandler<HTMLDivElement> | ||
onMouseDown | MouseEventHandler<HTMLDivElement> | ||
onMouseLeave | MouseEventHandler<HTMLDivElement> | ||
onMouseUp | MouseEventHandler<HTMLDivElement> | ||
onRelease | EventHandler<SyntheticEvent<HTMLDivElement, Event>> | Callback that's triggered after click i.e. mouseup and keyup of enter or space. | |
onTouchEnd | TouchEventHandler<HTMLDivElement> | ||
onTouchStart | TouchEventHandler<HTMLDivElement> | ||
radius | string | ||
size | IconButtonSizes | ||
tooltip | ReactNode | ||
tooltipProps | Omit<TooltipProps, "title" | "children"> | ||
variant | IconButtonVariants |
Prop name | Type | Default | Description |
---|---|---|---|
as | any | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. | |
autoComplete | "on" | "off" | "nope" | ||
defaultValue | string | @deprecated use initialValue | |
disabled | boolean | ||
fullWidth | boolean | width: 100% is too troublesome to handle with custom css because of the nesting | |
initialValue | string | ||
invalid | boolean | ||
leftElement | ReactNode | ||
leftElementContainerProps | HTMLAttributes<HTMLDivElement> & { ref?: MutableRefObject<HTMLDivElement>; } | ||
onChange | (e: ChangeEvent<HTMLInputElement>) => void | ||
onTextChange | (val: string) => void | ||
placeholder | ReactNode | ||
readOnly | boolean | ||
rightElement | ReactNode | <TimePickerIcon /> | |
rightElementContainerProps | HTMLAttributes<HTMLDivElement> & { ref?: MutableRefObject<HTMLDivElement>; } | ||
type | "number" | "text" | "password" | ||
value | string | ||
wrapperProps | WrapperProps |
Prop name | Type | Default | Description |
---|---|---|---|
as | any | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. | |
header | ReactNode | ||
headerProps | HeaderProps | ||
index | number | ||
initialValue | OptionValue | ||
onChange | (value: OptionValue) => void | ||
scrollerProps | TimePickerScrollerProps & { ref?: Ref<HTMLDivElement>; } | ||
value | OptionValue |
Prop name | Type | Default | Description |
---|---|---|---|
as | any | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. |
Prop name | Type | Default | Description |
---|---|---|---|
as | any | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. | |
header | ReactNode | Minute | |
headerProps | HeaderProps | ||
index | number | ||
scrollerProps | TimePickerScrollerProps & { ref?: Ref<HTMLDivElement>; } |
Prop name | Type | Default | Description |
---|---|---|---|
as | any | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. | |
disabled | boolean | Equivalent to button's disabled property. | |
index | number | ||
keyboardClickKeys | string[] | ||
onBlur | FocusEventHandler<HTMLDivElement> | ||
onClick | MouseEventHandler<HTMLDivElement> | ||
onKeyDown | KeyboardEventHandler<HTMLDivElement> | ||
onKeyUp | KeyboardEventHandler<HTMLDivElement> | ||
onMouseDown | MouseEventHandler<HTMLDivElement> | ||
onMouseLeave | MouseEventHandler<HTMLDivElement> | ||
onMouseUp | MouseEventHandler<HTMLDivElement> | ||
onRelease | EventHandler<SyntheticEvent<HTMLDivElement, Event>> | Callback that's triggered after click i.e. mouseup and keyup of enter or space. | |
onTouchEnd | TouchEventHandler<HTMLDivElement> | ||
onTouchStart | TouchEventHandler<HTMLDivElement> | ||
value | OptionValue |
Prop name | Type | Default | Description |
---|---|---|---|
arrow | ArrowProps | ||
as | any | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. | |
container | HTMLElement | ||
focusOnOpen | boolean | ||
immediate | boolean | ||
offset | [number, number] | ||
onComponentBlur | () => void | Callback function that will be called when the whole Balloon component is blurred. | |
placement | Placements | bottom-start | |
popperOptions | PopperPopperOptions | Options for floating.ui instance | |
popperUpdateKey | string | ||
shouldKeyDownReturn | (e: KeyboardEvent<HTMLDivElement>) => boolean | By default when the focus is within the balloon content, press any keys other than esc will move the focus back to the trigger element. Use this prop if you want to change this behavior, the boolean returned from this function will decide if the key down event should return the focus to trigger element. | |
springConfig | Partial<AnimationConfig> |
Prop name | Type | Default | Description |
---|---|---|---|
as | any | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. | |
header | ReactNode | Second | |
headerProps | HeaderProps | ||
index | number | ||
scrollerProps | TimePickerScrollerProps & { ref?: Ref<HTMLDivElement>; } |
Copyright © 2018-2025 Sea Labs