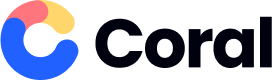
Coral is a thoroughly developed design system widely adopted by developers and designers for creating beautiful and user-friendly Sea internal products.
Copyright © 2018-2025 Sea Labs
Prop name | Type | Default | Description |
---|---|---|---|
clearInvalidInputOnBlur | boolean | true | Set to true to automatically clear input when the whole component is blurred. |
date | Dayjs | Controlled current calendar date, month, or year. This prop is also used to manage focus when using keyboard arrow navigation. | |
dateFormat | string | YYYY/MM/DD | Dayjs format to display from selected date, and parse input to autoselect date when the parse is valid. |
disabled | boolean | ||
displayDate | (value: Dayjs) => string | (value: Dayjs | null) => (value ? value.format(dateFormat) : '') | Callback to convert value to inputValue |
initialDate | Dayjs | Uncontrolled current calendar date, month, or year. This prop's default depends on value initialized. | |
initialInputValue | string | Uncontrolled input value. In this component, normally you would want to use controlled inputValue instead. | |
initialIsOpen | boolean | Uncontrolled of popup open state. | |
initialMode | CalendarModes | date | Uncontrolled calendar mode. |
initialValue | Dayjs | Uncontrolled selected date. In this component, normally you would want to use controlled value instead. | |
inputElement | ReactElement<any, any> | <DatePickerInput /> | Customizing input. Normally, how you use this prop is passing DatePickerInput with some additional props. |
inputValue | string | Controlled input value. You can think of this prop as text representation of value using dateFormat. | |
isDateDisabled | (date: Dayjs) => boolean | () => false | Whether a certain date cannot be selected. This prop is not only used to disable dates on the calendar, but also for input not to trigger selection when input is a valid date but disabled. |
isMonthDisabled | (date: Dayjs) => boolean | () => false | Disable input, date selection, and opening popup. |
isOpen | boolean | Controlled of popup open state. | |
isYearDisabled | (date: Dayjs) => boolean | () => false | |
mode | CalendarModes | Controlled calendar mode. | |
onChange | (value: Dayjs) => void | Callback when selected date changes. Note that this is different from onSelect because onChange is not triggered when the currently selected date is selected. | |
onDateChange | (date: Dayjs) => void | Callback when current calendar date changes.Generally, this prop is triggered when: (1) Navigating calendar using arrow next/previous month, year, or decade. (2) Keyboard date navigation. (3) Input changes that's valid. (4) Opening the popup. | |
onInputChange | (inputValue: string) => void | Callback when input value changes.Generally, it's triggered by: (1) Typing on the input. (2) Blur or clicking away from input, which will reset to selected value. (3) A date is selected. | |
onIsOpenChange | (isOpen: boolean) => void | Callback when popup open state changes.The event that triggers opening the popup is the same as Balloon component's - either click or keyboard arrow down. Closing is triggered when a date is selected. | |
onModeChange | (mode: CalendarModes) => void | Callback when calendar mode changes. | |
onSelect | (value: Dayjs) => void | Callback when a date is selected. Note that this is different from onChange because onSelect is triggered even when the currently selected date is selected again. | |
parseDate | (inputValue: string) => Dayjs | (value: string) => dayjs(value, dateFormat, true) | Callback to convert inputValue to value |
popupElement | ReactElement<any, any> | <DatePickerPopup /> | Customizing popup. Normally, how you use this prop is passing DatePickerPopup with some additional props. |
today | Dayjs | dayjs() | Can be used to customize current day. Helpful for testing to keep the result consistent. |
value | Dayjs | Controlled selected date. Pass null to indicate no date is selected, but still controlled. |
Prop name | Type | Default | Description |
---|---|---|---|
as | any | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. | |
height | number |
Prop name | Type | Default | Description |
---|---|---|---|
as | any | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. | |
date | Dayjs | ||
dateFooter | ReactNode | ||
dateHeader | ReactNode | ||
dateViewProps | ViewProps | ||
immediate | boolean | ||
initialDate | Dayjs | ||
initialMode | CalendarModes | ||
mode | CalendarModes | ||
monthFooter | ReactNode | ||
monthHeader | ReactNode | ||
monthViewProps | ViewProps | ||
onDateChange | (date: Dayjs) => void | ||
onModeChange | (mode: CalendarModes) => void | ||
onPreviousModeChange | (mode: CalendarModes) => void | ||
previousMode | CalendarModes | ||
renderDate | (props: RenderCalendarDateOptions, defaultCell: ReactElement<CalendarDateCellProps, any>) => ReactNode | (props: RenderCalendarDateOptions) => <DatePickerDateCell {...props} /> | |
renderDay | (props: RenderDayOptions, defaultCell: ReactElement<RenderDayOptions, any>) => ReactNode | ||
renderMonth | (props: RenderCalendarMonthOptions, defaultCell: ReactElement<CalendarMonthCellProps, any>) => ReactNode | (props: RenderCalendarMonthOptions) => <DatePickerMonthCell {...props} /> | |
renderYear | (props: RenderCalendarYearOptions, defaultCell: ReactElement<CalendarYearCellProps, any>) => ReactNode | (props: RenderCalendarYearOptions) => <DatePickerYearCell {...props} /> | |
startOfWeek | Day | Accepts numbers from 0 (Sunday) to 6 (Saturday). | |
today | Dayjs | ||
yearFooter | ReactNode | ||
yearHeader | ReactNode | ||
yearViewProps | ViewProps |
Prop name | Type | Default | Description |
---|---|---|---|
as | any | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. | |
autoFocus | boolean | ||
closeOnClick | boolean | true | |
date | Dayjs | ||
disableGrid | boolean | ||
disabled | boolean | Equivalent to button's disabled property. | |
keyboardClickKeys | string[] | ||
onBlur | FocusEventHandler<HTMLDivElement> | ||
onClick | MouseEventHandler<HTMLDivElement> | ||
onGridFocus | () => void | ||
onKeyDown | KeyboardEventHandler<HTMLDivElement> | ||
onKeyUp | KeyboardEventHandler<HTMLDivElement> | ||
onMouseDown | MouseEventHandler<HTMLDivElement> | ||
onMouseLeave | MouseEventHandler<HTMLDivElement> | ||
onMouseUp | MouseEventHandler<HTMLDivElement> | ||
onRelease | EventHandler<SyntheticEvent<HTMLDivElement, Event>> | Callback that's triggered after click i.e. mouseup and keyup of enter or space. | |
onTouchEnd | TouchEventHandler<HTMLDivElement> | ||
onTouchStart | TouchEventHandler<HTMLDivElement> | ||
preventScroll | boolean | ||
range | Range | ||
startOfWeek | Day | ||
today | Dayjs | ||
type | DateTypes | ||
variant | CellVariants |
Prop name | Type | Default | Description |
---|---|---|---|
as | any | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. |
Prop name | Type | Default | Description |
---|---|---|---|
as | void | WebTarget | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. | |
forwardedAs | void | WebTarget | ||
theme | DefaultTheme |
Prop name | Type | Default | Description |
---|---|---|---|
as | any | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. | |
autoComplete | "on" | "off" | "nope" | ||
autoformat | boolean | ||
clearable | boolean | ||
defaultValue | string | @deprecated use initialValue | |
disabled | boolean | ||
fullWidth | boolean | width: 100% is too troublesome to handle with custom css because of the nesting | |
initialValue | string | ||
invalid | boolean | ||
leftElement | ReactNode | ||
leftElementContainerProps | HTMLAttributes<HTMLDivElement> & { ref?: MutableRefObject<HTMLDivElement>; } | ||
onChange | (e: ChangeEvent<HTMLInputElement>) => void | ||
onTextChange | (val: string) => void | ||
placeholder | ReactNode | ||
readOnly | boolean | ||
rightElement | ReactNode | ||
rightElementContainerProps | HTMLAttributes<HTMLDivElement> & { ref?: MutableRefObject<HTMLDivElement>; } | ||
type | "number" | "text" | "password" | ||
value | string | ||
wrapperProps | WrapperProps |
Prop name | Type | Default | Description |
---|---|---|---|
as | any | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. | |
autoFocus | boolean | ||
disableDateMode | boolean | ||
disableGrid | boolean | ||
disabled | boolean | Equivalent to button's disabled property. | |
keyboardClickKeys | string[] | ||
month | Dayjs | ||
onBlur | FocusEventHandler<HTMLDivElement> | ||
onClick | MouseEventHandler<HTMLDivElement> | ||
onGridFocus | () => void | ||
onKeyDown | KeyboardEventHandler<HTMLDivElement> | ||
onKeyUp | KeyboardEventHandler<HTMLDivElement> | ||
onMouseDown | MouseEventHandler<HTMLDivElement> | ||
onMouseLeave | MouseEventHandler<HTMLDivElement> | ||
onMouseUp | MouseEventHandler<HTMLDivElement> | ||
onRelease | EventHandler<SyntheticEvent<HTMLDivElement, Event>> | Callback that's triggered after click i.e. mouseup and keyup of enter or space. | |
onTouchEnd | TouchEventHandler<HTMLDivElement> | ||
onTouchStart | TouchEventHandler<HTMLDivElement> | ||
preventScroll | boolean | ||
range | Range | ||
today | Dayjs | ||
variant | CellVariants |
Prop name | Type | Default | Description |
---|---|---|---|
arrow | ArrowProps | ||
as | any | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. | |
container | HTMLElement | ||
focusOnOpen | boolean | ||
immediate | boolean | ||
offset | [number, number] | [0, 1] | |
onComponentBlur | () => void | Callback function that will be called when the whole Balloon component is blurred. | |
placement | Placements | bottom-start | |
popperOptions | PopperPopperOptions | Options for Popper.js instance | |
popperUpdateKey | string | ||
shouldKeyDownReturn | (e: KeyboardEvent<HTMLDivElement>) => boolean | ||
springConfig | Partial<AnimationConfig> |
Prop name | Type | Default | Description |
---|---|---|---|
as | any | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. | |
closeOnClick | boolean | ||
colorType | ColorTypes | neuBg2nd | Set color based on theme. If color is specified, that prop is used instead of this. |
date | Dayjs | ||
disabled | boolean | Equivalent to button's disabled property. | |
keyboardClickKeys | string[] | ||
leftIcon | ReactNode | Element before the label. Equivalent to element on the label with some margin. | |
loading | boolean | ||
onBlur | FocusEventHandler<HTMLDivElement> | ||
onClick | MouseEventHandler<HTMLDivElement> | ||
onKeyDown | KeyboardEventHandler<HTMLDivElement> | ||
onKeyUp | KeyboardEventHandler<HTMLDivElement> | ||
onMouseDown | MouseEventHandler<HTMLDivElement> | ||
onMouseLeave | MouseEventHandler<HTMLDivElement> | ||
onMouseUp | MouseEventHandler<HTMLDivElement> | ||
onRelease | EventHandler<SyntheticEvent<HTMLDivElement, Event>> | Callback that's triggered after click i.e. mouseup and keyup of enter or space. | |
onTouchEnd | TouchEventHandler<HTMLDivElement> | ||
onTouchStart | TouchEventHandler<HTMLDivElement> | ||
radius | number | ||
rightIcon | ReactNode | Element after the label. Equivalent to element on the label with some margin. | |
size | ButtonSizes | ||
variant | ButtonVariants | Prefers naming it as variant because type is a known button html property. |
Prop name | Type | Default | Description |
---|---|---|---|
as | any | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. | |
autoFocus | boolean | ||
disableGrid | boolean | ||
disabled | boolean | Equivalent to button's disabled property. | |
keyboardClickKeys | string[] | ||
onBlur | FocusEventHandler<HTMLDivElement> | ||
onClick | MouseEventHandler<HTMLDivElement> | ||
onGridFocus | () => void | ||
onKeyDown | KeyboardEventHandler<HTMLDivElement> | ||
onKeyUp | KeyboardEventHandler<HTMLDivElement> | ||
onMouseDown | MouseEventHandler<HTMLDivElement> | ||
onMouseLeave | MouseEventHandler<HTMLDivElement> | ||
onMouseUp | MouseEventHandler<HTMLDivElement> | ||
onRelease | EventHandler<SyntheticEvent<HTMLDivElement, Event>> | Callback that's triggered after click i.e. mouseup and keyup of enter or space. | |
onTouchEnd | TouchEventHandler<HTMLDivElement> | ||
onTouchStart | TouchEventHandler<HTMLDivElement> | ||
preventScroll | boolean | ||
range | Range | ||
today | Dayjs | ||
type | YearTypes | ||
variant | CellVariants | ||
year | Dayjs |
Prop name | Type | Default | Description |
---|---|---|---|
clearInvalidInputOnBlur | boolean | Set to true to automatically clear input when the whole component is blurred. | |
date | Dayjs | Controlled current calendar date, month, or year. This prop is also used to manage focus when using keyboard arrow navigation. | |
dateFormat | string | YYYY/MM | Dayjs format to display from selected date, and parse input to autoselect date when the parse is valid. |
disabled | boolean | ||
displayDate | (value: Dayjs) => string | Callback to convert value to inputValue | |
initialDate | Dayjs | Uncontrolled current calendar date, month, or year. This prop's default depends on value initialized. | |
initialInputValue | string | Uncontrolled input value. In this component, normally you would want to use controlled inputValue instead. | |
initialIsOpen | boolean | Uncontrolled of popup open state. | |
initialMode | CalendarModes | month | Uncontrolled calendar mode. |
initialValue | Dayjs | Uncontrolled selected date. In this component, normally you would want to use controlled value instead. | |
inputElement | ReactElement<any, any> | Customizing input. Normally, how you use this prop is passing DatePickerInput with some additional props. | |
inputValue | string | Controlled input value. You can think of this prop as text representation of value using dateFormat. | |
isMonthDisabled | (month: Dayjs) => boolean | () => false | Disable input, date selection, and opening popup. |
isOpen | boolean | Controlled of popup open state. | |
isYearDisabled | (date: Dayjs) => boolean | () => false | |
mode | "month" | "year" | Controlled calendar mode. | |
onChange | (value: Dayjs) => void | Callback when selected date changes. Note that this is different from onSelect because onChange is not triggered when the currently selected date is selected. | |
onDateChange | (date: Dayjs) => void | Callback when current calendar date changes.Generally, this prop is triggered when: (1) Navigating calendar using arrow next/previous month, year, or decade. (2) Keyboard date navigation. (3) Input changes that's valid. (4) Opening the popup. | |
onInputChange | (inputValue: string) => void | Callback when input value changes.Generally, it's triggered by: (1) Typing on the input. (2) Blur or clicking away from input, which will reset to selected value. (3) A date is selected. | |
onIsOpenChange | (isOpen: boolean) => void | Callback when popup open state changes.The event that triggers opening the popup is the same as Balloon component's - either click or keyboard arrow down. Closing is triggered when a date is selected. | |
onModeChange | (mode: CalendarModes) => void | Callback when calendar mode changes. | |
onSelect | (value: Dayjs) => void | Callback when a date is selected. Note that this is different from onChange because onSelect is triggered even when the currently selected date is selected again. | |
parseDate | (inputValue: string) => Dayjs | Callback to convert inputValue to value | |
popupElement | ReactElement<any, any> | <MonthPickerPopup /> | Customizing popup. Normally, how you use this prop is passing DatePickerPopup with some additional props. |
today | Dayjs | Can be used to customize current day. Helpful for testing to keep the result consistent. | |
value | Dayjs | Controlled selected date. Pass null to indicate no date is selected, but still controlled. |
Prop name | Type | Default | Description |
---|---|---|---|
as | any | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. | |
date | Dayjs | ||
dateFooter | ReactNode | ||
dateViewProps | ViewProps | ||
immediate | boolean | ||
initialDate | Dayjs | ||
initialMode | CalendarModes | ||
mode | CalendarModes | ||
monthFooter | ReactNode | ||
monthHeader | ReactNode | ||
monthViewProps | ViewProps | ||
onDateChange | (date: Dayjs) => void | ||
onModeChange | (mode: CalendarModes) => void | ||
onPreviousModeChange | (mode: CalendarModes) => void | ||
previousMode | CalendarModes | ||
renderMonth | (props: RenderCalendarMonthOptions, defaultCell: ReactElement<CalendarMonthCellProps, any>) => ReactNode | (props: RenderCalendarMonthOptions) => <MonthPickerMonthCell {...props} /> | |
renderYear | (props: RenderCalendarYearOptions, defaultCell: ReactElement<CalendarYearCellProps, any>) => ReactNode | ||
startOfWeek | Day | Accepts numbers from 0 (Sunday) to 6 (Saturday). | |
today | Dayjs | ||
yearFooter | ReactNode | ||
yearHeader | ReactNode | ||
yearViewProps | ViewProps |
Prop name | Type | Default | Description |
---|---|---|---|
as | any | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. | |
autoFocus | boolean | ||
closeOnClick | boolean | true | |
disableDateMode | boolean | ||
disableGrid | boolean | ||
disabled | boolean | Equivalent to button's disabled property. | |
keyboardClickKeys | string[] | ||
month | Dayjs | ||
onBlur | FocusEventHandler<HTMLDivElement> | ||
onClick | MouseEventHandler<HTMLDivElement> | ||
onGridFocus | () => void | ||
onKeyDown | KeyboardEventHandler<HTMLDivElement> | ||
onKeyUp | KeyboardEventHandler<HTMLDivElement> | ||
onMouseDown | MouseEventHandler<HTMLDivElement> | ||
onMouseLeave | MouseEventHandler<HTMLDivElement> | ||
onMouseUp | MouseEventHandler<HTMLDivElement> | ||
onRelease | EventHandler<SyntheticEvent<HTMLDivElement, Event>> | Callback that's triggered after click i.e. mouseup and keyup of enter or space. | |
onTouchEnd | TouchEventHandler<HTMLDivElement> | ||
onTouchStart | TouchEventHandler<HTMLDivElement> | ||
preventScroll | boolean | ||
range | Range | ||
today | Dayjs | ||
variant | CellVariants |
Prop name | Type | Default | Description |
---|---|---|---|
arrow | ArrowProps | ||
as | any | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. | |
container | HTMLElement | ||
focusOnOpen | boolean | ||
immediate | boolean | ||
offset | [number, number] | ||
onComponentBlur | () => void | Callback function that will be called when the whole Balloon component is blurred. | |
placement | Placements | ||
popperOptions | PopperPopperOptions | Options for Popper.js instance | |
popperUpdateKey | string | ||
shouldKeyDownReturn | (e: KeyboardEvent<HTMLDivElement>) => boolean | ||
springConfig | Partial<AnimationConfig> |
Prop name | Type | Default | Description |
---|---|---|---|
clearInvalidInputOnBlur | boolean | Set to true to automatically clear input when the whole component is blurred. | |
date | Dayjs | Controlled current calendar date, month, or year. This prop is also used to manage focus when using keyboard arrow navigation. | |
dateFormat | string | YYYY | Dayjs format to display from selected date, and parse input to autoselect date when the parse is valid. |
disabled | boolean | ||
displayDate | (value: Dayjs) => string | Callback to convert value to inputValue | |
initialDate | Dayjs | Uncontrolled current calendar date, month, or year. This prop's default depends on value initialized. | |
initialInputValue | string | Uncontrolled input value. In this component, normally you would want to use controlled inputValue instead. | |
initialIsOpen | boolean | Uncontrolled of popup open state. | |
initialValue | Dayjs | Uncontrolled selected date. In this component, normally you would want to use controlled value instead. | |
inputElement | ReactElement<any, any> | Customizing input. Normally, how you use this prop is passing DatePickerInput with some additional props. | |
inputValue | string | Controlled input value. You can think of this prop as text representation of value using dateFormat. | |
isMonthDisabled | (date: Dayjs) => boolean | Disable input, date selection, and opening popup. | |
isOpen | boolean | Controlled of popup open state. | |
isYearDisabled | (month: Dayjs) => boolean | () => false | |
onChange | (value: Dayjs) => void | Callback when selected date changes. Note that this is different from onSelect because onChange is not triggered when the currently selected date is selected. | |
onDateChange | (date: Dayjs) => void | Callback when current calendar date changes.Generally, this prop is triggered when: (1) Navigating calendar using arrow next/previous month, year, or decade. (2) Keyboard date navigation. (3) Input changes that's valid. (4) Opening the popup. | |
onInputChange | (inputValue: string) => void | Callback when input value changes.Generally, it's triggered by: (1) Typing on the input. (2) Blur or clicking away from input, which will reset to selected value. (3) A date is selected. | |
onIsOpenChange | (isOpen: boolean) => void | Callback when popup open state changes.The event that triggers opening the popup is the same as Balloon component's - either click or keyboard arrow down. Closing is triggered when a date is selected. | |
onSelect | (value: Dayjs) => void | Callback when a date is selected. Note that this is different from onChange because onSelect is triggered even when the currently selected date is selected again. | |
parseDate | (inputValue: string) => Dayjs | Callback to convert inputValue to value | |
popupElement | ReactElement<any, any> | <YearPickerPopup /> | Customizing popup. Normally, how you use this prop is passing DatePickerPopup with some additional props. |
today | Dayjs | Can be used to customize current day. Helpful for testing to keep the result consistent. | |
value | Dayjs | Controlled selected date. Pass null to indicate no date is selected, but still controlled. |
Prop name | Type | Default | Description |
---|---|---|---|
as | any | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. | |
date | Dayjs | ||
dateFooter | ReactNode | ||
dateViewProps | ViewProps | ||
immediate | boolean | ||
initialDate | Dayjs | ||
initialMode | CalendarModes | ||
mode | CalendarModes | ||
monthFooter | ReactNode | ||
monthViewProps | ViewProps | ||
onDateChange | (date: Dayjs) => void | ||
onModeChange | (mode: CalendarModes) => void | ||
onPreviousModeChange | (mode: CalendarModes) => void | ||
previousMode | CalendarModes | ||
renderYear | (props: RenderCalendarYearOptions, defaultCell: ReactElement<CalendarYearCellProps, any>) => ReactNode | (props: RenderCalendarYearOptions) => <YearPickerYearCell {...props} /> | |
startOfWeek | Day | Accepts numbers from 0 (Sunday) to 6 (Saturday). | |
today | Dayjs | ||
yearFooter | ReactNode | ||
yearHeader | ReactNode | ||
yearViewProps | ViewProps |
Prop name | Type | Default | Description |
---|---|---|---|
arrow | ArrowProps | ||
as | any | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. | |
container | HTMLElement | ||
focusOnOpen | boolean | ||
immediate | boolean | ||
offset | [number, number] | ||
onComponentBlur | () => void | Callback function that will be called when the whole Balloon component is blurred. | |
placement | Placements | ||
popperOptions | PopperPopperOptions | Options for Popper.js instance | |
popperUpdateKey | string | ||
shouldKeyDownReturn | (e: KeyboardEvent<HTMLDivElement>) => boolean | ||
springConfig | Partial<AnimationConfig> |
Prop name | Type | Default | Description |
---|---|---|---|
as | any | Tag or component, but a simple tag (i.e. built-in components, e.g. div, span) is recommended. | |
autoFocus | boolean | ||
closeOnClick | boolean | true | |
disableGrid | boolean | ||
disabled | boolean | Equivalent to button's disabled property. | |
keyboardClickKeys | string[] | ||
onBlur | FocusEventHandler<HTMLDivElement> | ||
onClick | MouseEventHandler<HTMLDivElement> | ||
onGridFocus | () => void | ||
onKeyDown | KeyboardEventHandler<HTMLDivElement> | ||
onKeyUp | KeyboardEventHandler<HTMLDivElement> | ||
onMouseDown | MouseEventHandler<HTMLDivElement> | ||
onMouseLeave | MouseEventHandler<HTMLDivElement> | ||
onMouseUp | MouseEventHandler<HTMLDivElement> | ||
onRelease | EventHandler<SyntheticEvent<HTMLDivElement, Event>> | Callback that's triggered after click i.e. mouseup and keyup of enter or space. | |
onTouchEnd | TouchEventHandler<HTMLDivElement> | ||
onTouchStart | TouchEventHandler<HTMLDivElement> | ||
preventScroll | boolean | ||
range | Range | ||
today | Dayjs | ||
type | YearTypes | ||
variant | CellVariants | ||
year | Dayjs |
Copyright © 2018-2025 Sea Labs